Default Attributes on Active Record Associations in Rails
I worked up a quick Active Record association extention that allows you to define default attributes on new records created through the association:
class User < ActiveRecord::Base
has_many :events, :extend => AssociationDefaultAttributes do
def default_attributes
{ :venue => proxy_owner.default_venue }
end
end
end
module AssociationDefaultAttributes
def create(attrs, &block)
super(default_attributes.merge(attrs), &block)
end
def build(attrs={}, &block)
super(default_attributes.merge(attrs), &block)
end
end
What this is doing is extending the association proxy
object
with a module that overrides the build
and create
methods such that they
use the default values returned by the default_attributes
method. Much
neater than setting them elsewhere, no?
I love this sort of meta-programming in Ruby, it’s so much nice than the way
we used to do it in the bad old days using alias_method_chain
.
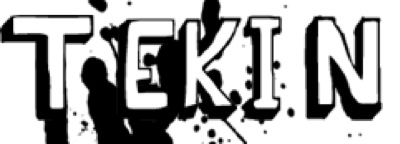